Mastering the Parallax Effect: A Guide to Using the React-scroll-parallax Library
Unlock dynamic website design with the React-scroll-parallax library.
An effect where elements or backgrounds move at different speeds while scrolling. It makes the website feel more alive & dynamic, which in return makes it more interesting for users to check out and stay on it.
There are multiple Parallax examples out there, and not just on the web. Like parallax errors with measuring instruments. When reading values on a measuring instrument face to face with the pointer and from the side, you get/read different values. It's because of the layered elements, you have the values & the pointer that aren't on the same plane.
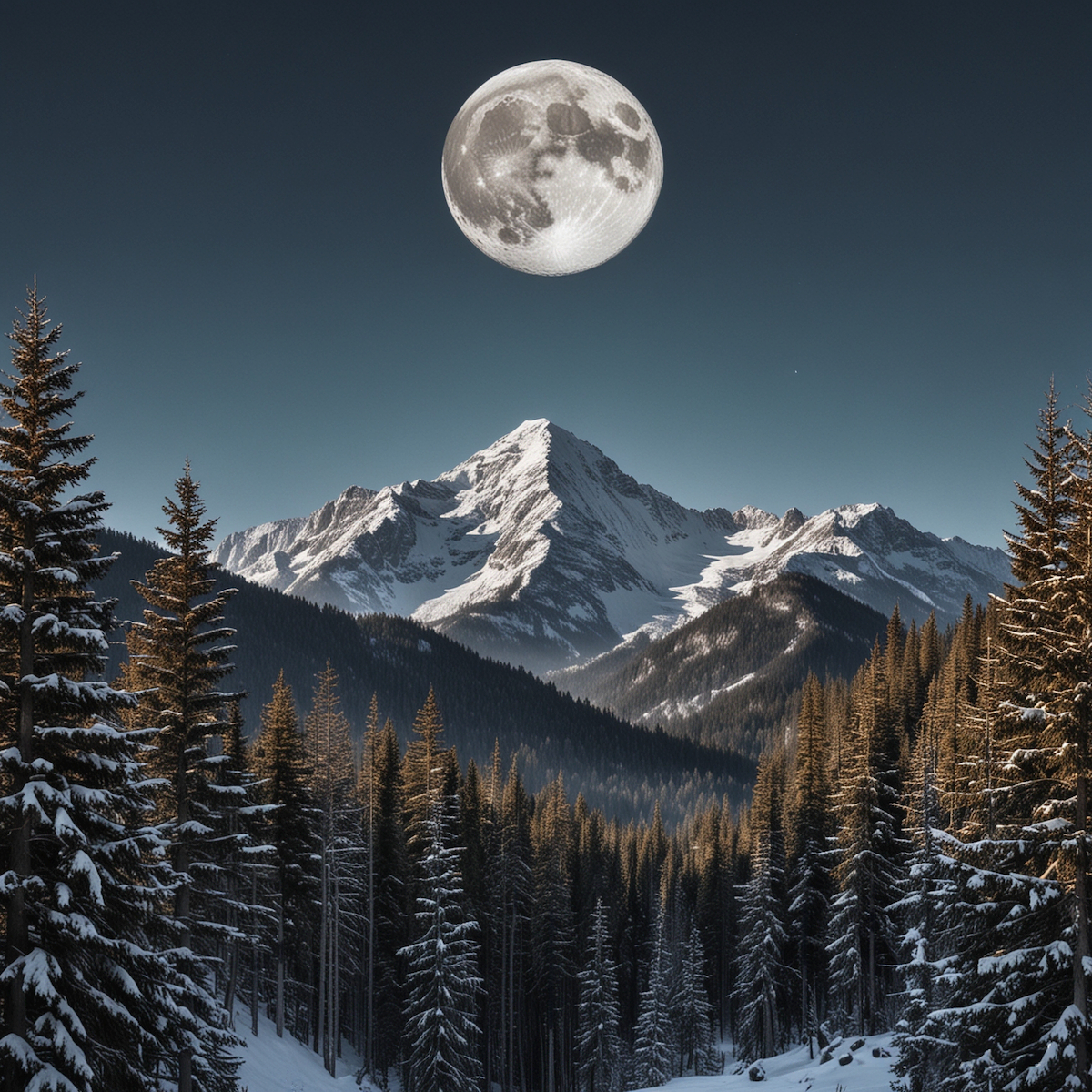
Parallax can also be found in cartoons and video games, where backgrounds are layered and they move at different speed creating the feel of depth. It's the same with the parallax on the web, background moves with different speeds creating beautiful, and dynamic feel.
It first came in 2011 on the Beercamp website and it became a trend ever since. Parallax effect can be found on many websites, some of them use simple parallax effects, other use parallax, but go more deeply into advanced scroll animations.
Websites such as:
- Firewatch game
- Web design and art history
- New York times: Snow falls
- Apple Macbook Air
- Sketch prototyping
Websites like Sketch use simple parallax to make static design feel dynamic, others, like apple use far more advanced scroll animations and smooth scrolls. In this blog we will go through simple parallax examples, and dive into some advanced scroll animations.
Why do we want it, what are the pros & cons of the parallax effect?
Here’s a quick breakdown of its pros and cons to consider when implementing the parallax effect in your project:
Pros:
- Enhanced Aesthetics: The primary advantage of the parallax effect is its ability to beautify a webpage. It transforms simple, static designs into dynamic experiences with minimal effort, infusing pages with a sense of depth and motion that can make them feel more alive and engaging.
- Increased Engagement: Parallax scrolling can direct the user's attention to specific elements for extended periods. Since elements move at varying speeds, it creates a more captivating experience, encouraging users to spend more time exploring the content.
- Improved User Experience: By providing a unique 3D feel and interactive storytelling capability, the parallax effect can make a website stand out. It offers a memorable way to present information, making the user experience more engaging and less monotonous.
Cons:
- Performance Issues: One of the main drawbacks of the parallax effect is its potential to negatively impact website performance. It can increase loading times and lead to choppy scrolling on less powerful devices or browsers, detracting from the user experience.
- SEO Impact: Parallax scrolling can present challenges for search engine optimization (SEO). Since content might be presented on a single page, it can be harder for search engines to index the site effectively, potentially reducing its visibility in search results.
- Usability Concerns: While visually appealing, parallax effects can sometimes make content harder to read or navigate, especially for users with disabilities. It can also disorient or distract users, leading to a less intuitive navigation experience.
- Overuse: Excessive use of parallax scrolling can overshadow the website's actual content, making it feel gimmicky or overwhelming rather than enhancing the user experience.
How to implement it?
Implementing a simple scroll parallax effect in a React application can indeed be straightforward, especially with the help of libraries designed to handle parallax effects.
First, you need a parallax library that works with React. Install react-scroll-parallax using npm or yarn:
npm install react-scroll-parallax
yarn add react-scroll-parallax
Here’s a basic outline of how you might proceed, using a react-scroll-parallax library for React. Note that the actual implementation details can vary based on the library you choose.
export default Layout: React.FC = () =>
<ParallaxProvider>{children}</ParallaxProvider>;
First, we wrap our layout component with the ParallaxProvider so that we can use parallax functionalities in our components
Simple Parallax
<div>
<h1>Simple parallax</h1>
<div>
<Parallax speed={10}>
<p>
The parallax effect refers to the apparent displacement or
difference in the position of an object when viewed from
different angles. This effect is often observed in everyday
life, such as when you view objects from different positions
with your eyes or when you move your head.
</p>
</Parallax>
<Parallax speed={50}>
<Image
src="/images/sofa.png"
alt="Sofa image"
width={600}
height={600}
/>
</Parallax>
</div>
</div>
Next, import the Parallax component and encapsulate the desired element for animation. By assigning the speed attribute to this component, you effortlessly achieve a straightforward scroll parallax effect.
In this example, the image moves more rapidly than the text, while the title remains stationary. We applied the speed attribute to both the text and the image, influencing the translateY or translateX property according to the scrolling direction.
Essentially, a speed of {10} corresponds to a movement of translateY: ['100px', '-100px'], indicating the element's vertical displacement from its original position.
Similarly, a negative value, such as speed={-10}, inverses this effect, resulting in translateY: ['-100px', '100px'].
Easing & scaling examples
<div>
<Parallax
translateX={[-40, 100, 'easeIn']}
translateY={[0, 120, 'easeIn']}
>
<Image
src="/images/scale/car.png"
alt="car"
width={400}
height={400}
/>
</Parallax>
<Parallax
translateX={[100, 0]}
translateY={[40, -100]}
>
<Image
src="/images/scale/motorcycle.png"
alt="motorcycle"
width={200}
height={200}
/>
</Parallax>
</div>
Additionally, you have the option to apply an easing property to the Parallax component.
Even if two images are set to move at the same speed, the easing effect will cause them to transition differently, as demonstrated in the accompanying video.
The mentioned example demonstrates effectiveness with a background image, allowing you to manipulate elements such as vehicles during scrolling to create interactive features effortlessly. Note that each animation requires slight adjustments to its values. Experimentation and fine-tuning are key to achieving the desired effect.
<div>
<div>
<Parallax scale={[0.9, 1.8]}>
<Image
src="/images/scale/tv.png"
alt="image"
width={500}
height={500}
/>
</Parallax>
<div>
<Parallax speed={10}>
<h2>Scale parallax</h2>
</Parallax>
</div>
</div>
Scaling is also achievable by assigning the scale, scaleZ, scaleX, or scaleY properties to the component.
This action results in elements being scaled as you scroll, offering an additional layer of interaction and visual dynamics to your page.
In the provided example, the image undergoes scaling from 0.9 to 1.8 times its original height and width as the page is scrolled.
This dynamic effect enhances the visual interaction, making the image more engaging as it adjusts in size based on user scroll behavior.
Advanced parallax effect
The video above shows us an example of layered background images that move at different speeds from each other. Creating a dimensional feeling when scrolling, and being visually appealing.
<ParallaxBanner>
<ParallaxBannerLayer speed={-60} style={{ zIndex: 0 }}>
<Image
src="/images/sky.png"
alt="Sky image"
/>
</ParallaxBannerLayer>
<ParallaxBannerLayer speed={-110} style={{ zIndex: 0 }}>
<Image
src="/images/moon.png"
alt="Moon image"
/>
</ParallaxBannerLayer>
<ParallaxBannerLayer speed={-50} style={{ zIndex: 1 }}>
<Image
src="/images/mountain.png"
alt="mountain image"
/>
</ParallaxBannerLayer>
<ParallaxBannerLayer speed={-40} style={{ zIndex: 3 }}>
<Image
src="/images/trees-3.png"
alt="trees image"
/>
</ParallaxBannerLayer>
<ParallaxBannerLayer speed={-50} style={{ zIndex: 2 }}>
<Image
src="/images/trees-2.png"
alt="trees image"
/>
</ParallaxBannerLayer>
<ParallaxBannerLayer speed={0} style={{ zIndex: 4 }}>
<Image
src="/images/trees-1.png"
alt="trees image"
/>
</ParallaxBannerLayer>
<ParallaxBannerLayer speed={-150} style={{ zIndex: 1 }}>
<h1 className="advanced-title">Advanced parallax</h1>
</ParallaxBannerLayer>
<ParallaxBannerLayer speed={-150} style={{ zIndex: 2 }}>
<h1 className="advanced-title-2">With banners</h1>
</ParallaxBannerLayer>
</ParallaxBanner>
You can see we used different components to achieve this effect here.
First, we wrapped the entire element with the ParallaxBanner component. This component enables us to use layered mages wrapped inside ParallaxBannerLayer. It's also possible to pass layers prop to the ParallaxBanner, so we don't have to use Layer components.
<ParallaxBanner
layers={[
{ image: '/images/sky.png', speed: -30 },
{ image: '/images/land.png', speed: 10 },
]}
/>
In the example shown, the results are the same.
In our example, both methods work the same. After using ParallaxBanner, wrap elements with ParallaxBannerLayer and adjust their speed properties to get the effect you want. Use the z-index property to make certain elements stand out by keeping them in front during scrolling.
Finishing thoughts
As shown and said above, parallax and scroll effects can be the best way to transform your website into a unique experience, that is visually appealing and interesting.
Parallax effects can make your website look great, but overdoing it can ruin the user experience. Use these effects sparingly and avoid animating every element on scroll.